With PaymentSheet, you can make payments in a single flow. As soon as the User presses the payment button, the payment is completed. (If you want user have some flow after that, please use
paymentFlow
method)
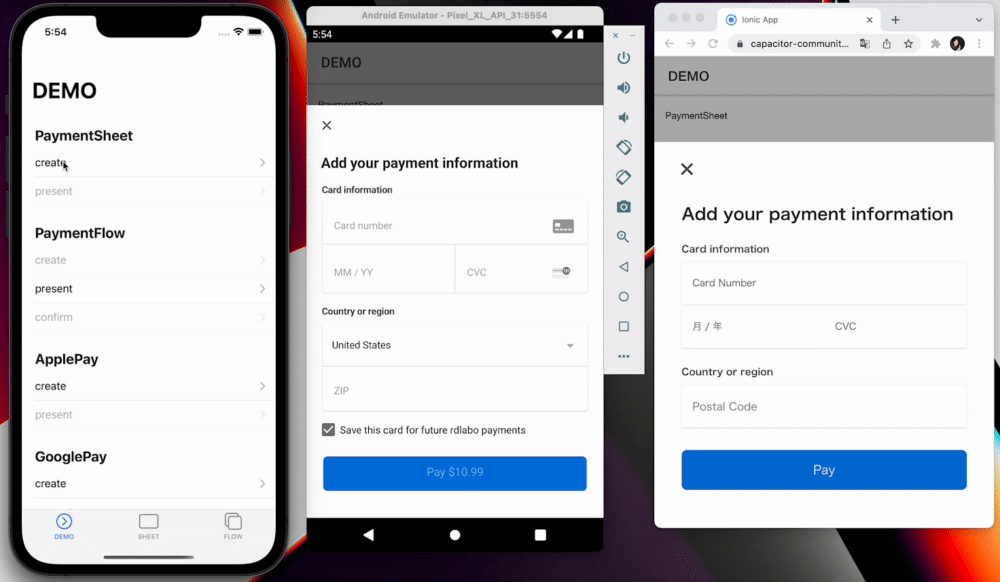
This method can be used for both immediate payment with
PaymentIntent
, and future payments with
SetupIntent
.
Don't know what these Intent is? Learn it first at the official Stripe website.
PaymentIntent:
https://stripe.com/docs/payments/payment-intentshttps://stripe.com/docs/payments/payment-intents
SetupIntent:
https://stripe.com/docs/payments/save-and-reuse?platform=webhttps://stripe.com/docs/payments/save-and-reuse?platform=web
This guide will show you an easy way to make instant payments.
🐾 Implements Guide
1. createPaymentSheet
This method is settings for PaymentSheet. Before use, you should connect to your backend endpoint, and get every key. This is "not" function at this Plugin. So you will use
HTTPClient
,
Axios
, Ajax
, and so on. Here is example of Angular HttpClient. This method will get
paymentIntent
,
ephemeralKey
, and ephemeralKey
.
Stripe provide how to implement backend:
https://stripe.com/docs/payments/accept-a-payment?platform=ios#add-server-endpointhttps://stripe.com/docs/payments/accept-a-payment?platform=ios#add-server-endpoint
After that, you set these key to createPaymentSheet
method.
import { Stripe, PaymentSheetEventsEnum } from '@capacitor-community/stripe';
(async () => {
const { paymentIntent, ephemeralKey, customer } = await this.http.post<{
paymentIntent: string;
ephemeralKey: string;
customer: string;
}>(environment.api + 'payment-sheet', {}).pipe(first()).toPromise(Promise);
await Stripe.createPaymentSheet({
paymentIntentClientSecret: paymentIntent,
customerId: customer,
customerEphemeralKeySecret: ephemeralKey,
});
})();
You can use options of CreatePaymentSheetOption
on
createPaymentSheet
.
method
createPaymentSheet(...)
createPaymentSheet(options: CreatePaymentSheetOption) => Promise<void>
Props paymentIntentClientSecret
, customerId
, customerEphemeralKeySecret
are
required. And be able to set style alwaysLight
or alwaysDark
, prepare
Apple Pay and GooglePay on PaymentSheet.
interface
CreatePaymentSheetOption
Prop | Type | Description | Default |
paymentIntentClientSecret | string |
Any documentation call 'paymentIntent' Set paymentIntentClientSecret or setupIntentClientSecret |
|
setupIntentClientSecret | string |
Any documentation call 'paymentIntent' Set paymentIntentClientSecret or setupIntentClientSecret |
|
billingDetailsCollectionConfiguration | BillingDetailsCollectionConfiguration |
Optional billingDetailsCollectionConfiguration | |
customerEphemeralKeySecret |
string | Any documentation call 'ephemeralKey' | |
customerId |
string | Any documentation call 'customer' | |
enableApplePay |
boolean | If you set payment method ApplePay, this set true | false |
applePayMerchantId | string | If set enableApplePay false, Plugin ignore here. |
|
enableGooglePay | boolean |
If you set payment method GooglePay, this set true | false |
GooglePayIsTesting |
boolean | | false, |
countryCode |
string | use ApplePay and GooglePay. If set enableApplePay and enableGooglePay false, Plugin ignore here. |
"US" |
merchantDisplayName | string |
| "App Name" |
returnURL |
string | | "" |
style |
'alwaysLight' | 'alwaysDark' | iOS Only | undefined |
withZipCode | boolean | Platform: Web only Show ZIP code field. |
true |
2. presentPaymentSheet
When you do presentPaymentSheet
method, plugin present PaymentSheet and get result. This method must do after
createPaymentSheet
.
(async () => {
const result = await Stripe.presentPaymentSheet();
if (result.paymentResult === PaymentSheetEventsEnum.Completed) {
}
})();
You can get PaymentSheetResultInterface
from
presentPaymentSheet
.
method
presentPaymentSheet()
presentPaymentSheet() => Promise<{ paymentResult: PaymentSheetResultInterface; }>
PaymentSheetResultInterface
is created from Enum of PaymentSheetEventsEnum
. So you should import and check result.
type alias
PaymentSheetResultInterface
PaymentSheetEventsEnum.Completed | PaymentSheetEventsEnum.Canceled | PaymentSheetEventsEnum.Failed
3. addListener
Method of PaymentSheet notify any listeners. If you want to get event of payment process is 'Completed', you should add
PaymentSheetEventsEnum.Completed
listener to
Stripe
object:
Stripe.addListener(PaymentSheetEventsEnum.Completed, () => {
console.log('PaymentSheetEventsEnum.Completed');
});
The event name you can use is
PaymentSheetEventsEnum
.
enum
PaymentSheetEventsEnum
Members | Value |
Loaded |
"paymentSheetLoaded" |
FailedToLoad |
"paymentSheetFailedToLoad" |
Completed |
"paymentSheetCompleted" |
Canceled |
"paymentSheetCanceled" |
Failed |
"paymentSheetFailed" |
📖 Reference
See the Stripe Documentation for more information. This plugin is wrapper, so there information seems useful for you.
Accept a payment(iOS)
This plugin use PaymentSheet on pod 'Stripe'
:
https://stripe.com/docs/payments/accept-a-payment?platform=ioshttps://stripe.com/docs/payments/accept-a-payment?platform=ios
Accept a payment(Android)
This plugin use PaymentSheet on com.stripe:stripe-android
:
https://stripe.com/docs/payments/accept-a-payment?platform=androidhttps://stripe.com/docs/payments/accept-a-payment?platform=android