With Apple Pay, you can make instant payments in a single flow. Please check settings:
https://stripe.com/docs/apple-payhttps://stripe.com/docs/apple-pay
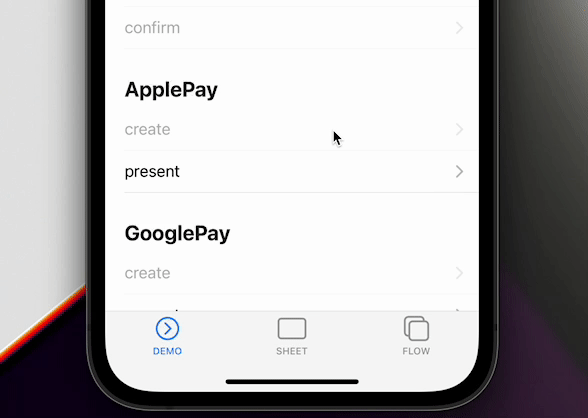
🐾 Implements Guide
Prepare settings
For using Apple Pay, you need some settings.
- Register for an Apple Merchant ID
- Create a new Apple Pay certificate
- Integrate with Xcode
Detail information is here:
https://stripe.com/docs/apple-pay#merchantidhttps://stripe.com/docs/apple-pay#merchantid
If these are not done correctly and are different from the options given to
createApplePay
, this method will not be able to run.
1. isApplePayAvailable
First, you should check to be able to use Apple Pay on device.
import { Stripe, ApplePayEventsEnum } from '@capacitor-community/stripe';
(async() => {
const isAvailable = Stripe.isApplePayAvailable().catch(() => undefined);
if (isAvailable === undefined) {
return;
}
})();
This method return resolve(): void
or
reject('Not implemented on Device.')
.
method
isApplePayAvailable()
isApplePayAvailable() => Promise<void>
2. createApplePay
You should connect to your backend endpoint, and get every key. This is "not" function at this Plugin. So you can use
HTTPClient
,
Axios
, Ajax
, and so on.
Stripe provide how to implement backend:
https://stripe.com/docs/payments/accept-a-payment?platform=ios#add-server-endpointhttps://stripe.com/docs/payments/accept-a-payment?platform=ios#add-server-endpoint
After that, you set these key to createApplePay
method.
(async() => {
const { paymentIntent } = await this.http.post<{
paymentIntent: string;
}>(environment.api + 'payment-sheet', {}).pipe(first()).toPromise(Promise);
await Stripe.createApplePay({
paymentIntentClientSecret: paymentIntent,
paymentSummaryItems: [{
label: 'Product Name',
amount: 1099.00
}],
merchantDisplayName: 'rdlabo',
countryCode: 'US',
currency: 'USD',
});
})();
method
createApplePay(...)
createApplePay(options: CreateApplePayOption) => Promise<void>
You can use options of CreateApplePayOption
on createApplePay
.
merchantIdentifier
must be the same as the value registered in Apple Developer Website.
interface
CreateApplePayOption
Prop | Type |
paymentIntentClientSecret |
string |
paymentSummaryItems | { label: string; amount: number; }[] |
merchantIdentifier | string |
countryCode | string |
currency |
string |
requiredShippingContactFields |
('postalAddress' | 'phoneNumber' | 'emailAddress' | 'name')[] |
allowedCountries |
string[] |
allowedCountriesErrorDescription |
string |
3. presentApplePay
present in
createApplePay
is single flow. You don't need to confirm method.
(async() => {
const result = await Stripe.presentApplePay();
if (result.paymentResult === ApplePayEventsEnum.Completed) {
}
})();
method
presentApplePay()
presentApplePay() => Promise<{ paymentResult: ApplePayResultInterface; }>
ApplePayResultInterface
is created from Enum of ApplePayEventsEnum
. So you should import and check result.
type alias
ApplePayResultInterface
ApplePayEventsEnum.Completed | ApplePayEventsEnum.Canceled | ApplePayEventsEnum.Failed | ApplePayEventsEnum.DidSelectShippingContact | ApplePayEventsEnum.DidCreatePaymentMethod
4. addListener
Method of Apple Pay notify any listeners. If you want to get event of payment process is 'Completed', you should add
ApplePayEventsEnum.Completed
listener to
Stripe
object:
Stripe.addListener(ApplePayEventsEnum.Completed, () => {
console.log('ApplePayEventsEnum.Completed');
});
The event name you can use is
ApplePayEventsEnum
.
enum
ApplePayEventsEnum
Members | Value |
Loaded |
"applePayLoaded" |
FailedToLoad |
"applePayFailedToLoad" |
Completed |
"applePayCompleted" |
Canceled |
"applePayCanceled" |
Failed | "applePayFailed" |
DidSelectShippingContact | "applePayDidSelectShippingContact" |
DidCreatePaymentMethod | "applePayDidCreatePaymentMethod" |
📖 Reference
See the Stripe Documentation for more information. This plugin is wrapper, so there information seems useful for you.
Apple Pay(iOS)
This plugin use STPApplePayContext on pod 'Stripe'
:
https://stripe.com/docs/apple-payhttps://stripe.com/docs/apple-pay
"Merchant name" on the apple pay payment sheet #115
This is good issue for setting "Merchant name".
https://github.com/capacitor-community/stripe/issues/115https://github.com/capacitor-community/stripe/issues/115